Logging Objects in Javascript
There seem to be three ways you can log out an object in Javascript and each gives you different results. A co-worker pointed out these differences to me so I thought I’d share that information here.
These objects are logged to the Chrome console
Let’s assume we have the following contribs object in scope:
const contribs = {
"data": {
"viewer": {
"login": "ssanj",
"contributedRepositories": {
"nodes": [
{
"name": "giter8"
},
{
"name": "scalacheck"
},
{
"name": "sbt-assembly"
},
{
"name": "package_control_channel"
},
{
"name": "dotty"
},
{
"name": "scapegoat"
},
{
"name": "website"
},
{
"name": "ssanj.github.io"
},
{
"name": "babyloncandle"
},
{
"name": "ensime.github.io"
}
]
}
}
}
};
Use toString
With
console.log(contribs.toString());
we get the following:
"[object Object]"
And this is not very useful. If you do see this output when you are trying to log out the contents of an object then choose on of the other two options mentioned below.
Use JSON.stringify
With
console.log(JSON.stringify(contribs));
we get:
"{"data":{"viewer":{"login":"ssanj","contributedRepositories":{"nodes":[{"name":"giter8"},{"name":"scalacheck"},{"name":"sbt-assembly"},{"name":"package_control_channel"},{"name":"dotty"},{"name":"scapegoat"},{"name":"website"},{"name":"ssanj.github.io"},{"name":"babyloncandle"},{"name":"ensime.github.io"}]}}}}"
While that is more useful than the toString() option, it would be nice if we can pretty print the same information. Luckily that functionality is built into JSON.stringify:
console.log(JSON.stringify(contribs, null, 2));
which results in:
"{
"data": {
"viewer": {
"login": "ssanj",
"contributedRepositories": {
"nodes": [
{
"name": "giter8"
},
{
"name": "scalacheck"
},
{
"name": "sbt-assembly"
},
{
"name": "package_control_channel"
},
{
"name": "dotty"
},
{
"name": "scapegoat"
},
{
"name": "website"
},
{
"name": "ssanj.github.io"
},
{
"name": "babyloncandle"
},
{
"name": "ensime.github.io"
}
]
}
}
}
}"
Use the object
Finally if we directly log the object:
console.log(contribs);
we get the Javascript object graph for contrib:
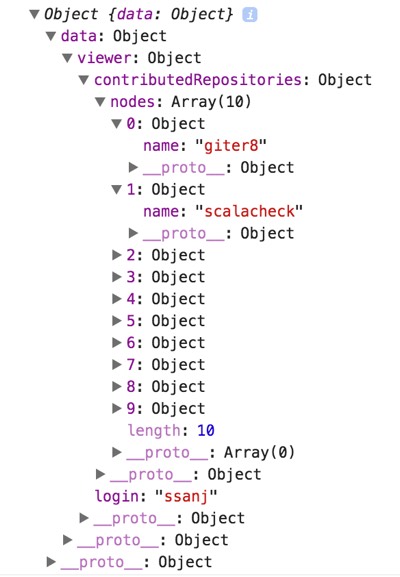
which we can then explore at our leisure.